The example defines a usage of a ListView to display distances in an
identification application.
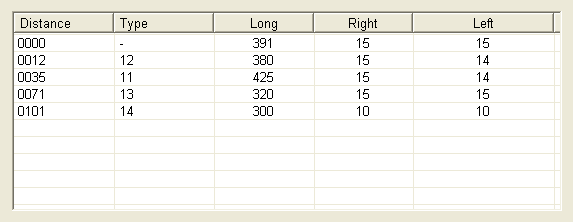
def CreateProductListView():
import CLR
import CLR.System.Windows.Forms as WinForms
from CLR.System.Drawing import Size, Point, ContentAlignment,Rectangle,Bitmap
# Load custom assemblies
from CLR.System.Reflection import Assembly
Assembly.LoadWithPartialName("OverlayPanel")
from CLR.Tordivel import OverlayPanel
class ProductListView(WinForms.ListView):
def __init__(self):
# This enables the panel to follow resizing of the parent
self.Dock = WinForms.DockStyle.None
true = 1
# Set the view to show details.
self.View = WinForms.View.Details;
# Allow the user to edit item text.
self.LabelEdit = 0;
# Allow the user to rearrange columns.
self.AllowColumnReorder = 0;
# Display check boxes.
self.CheckBoxes = 0;
# Select the item and subitems when selection is made.
self.FullRowSelect = true;
# Display grid lines.
self.GridLines = true;
# Sort the items in the list in ascending order.
self.Sorting = WinForms.SortOrder.Ascending;
# Create columns for the items and subitems.
self.Columns.Add("Distance", 100, WinForms.HorizontalAlignment.Center);
self.Columns.Add("Type", 100, WinForms.HorizontalAlignment.Left);
self.Columns.Add("Long", 100, WinForms.HorizontalAlignment.Center);
self.Columns.Add("Right", 99, WinForms.HorizontalAlignment.Center);
self.Columns.Add("Left", 99, WinForms.HorizontalAlignment.Center);
def Clear(self):
self.Items.Clear()
def AddProduct(self,products):
item = WinForms.ListViewItem(products);
self.Items.AddRange([item])
print products
return ProductListView()
Example 1: Add Products to ListView
def distance(self,dictionary,key,left,right,long):
def padspaces(d):
if d < 10:
return '000'+str(int(d))
if d < 100:
return '00'+str(int(d))
if d < 1000:
return '0'+str(int(d))
else :
return str(int(d))
dict = dictionary[key]
d = abs(dict['right']-right)+abs(dict['left']-left)+abs(dict['long']-long)
self.productSelection.listView.AddProduct([padspaces(d),str(key),str(dict['long']),str(dict['right']),str(dict['left'])])
return d
|